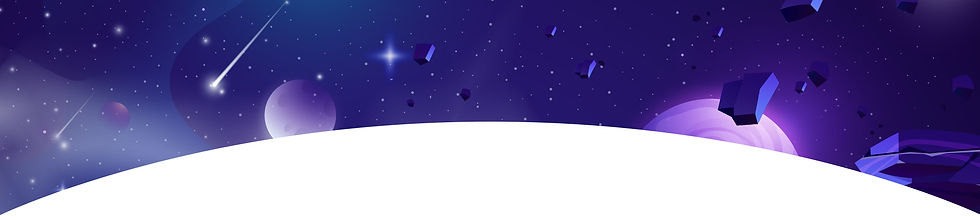
C++ 101
High-Level Language | Text Based | Age 14-18 | 56 Lessons
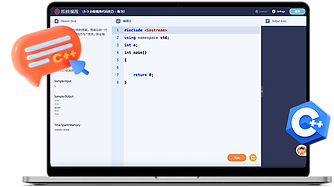
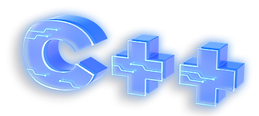
C++ is an object-oriented programming (OOP) language that is viewed by many as the best language for creating large-scale applications. In C++, programmers will start as beginners in C++.
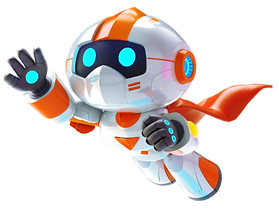
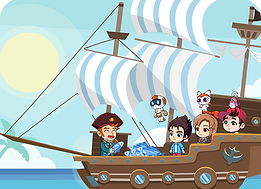.png)
Unit 01
7 Lessons
133 Challenges
1.Learn the basic structure of C++ programs and the application of the output command 'cout'.
2.Study integer variables and the application of variable input.
3.Review the use of integer variables and variable input, learn the 'if' statement for conditional checks, and understand relational operators.
4.Review the 'if' statement, learn the 'for' loop statement, and master repeating code execution with 'for' loops.
5.Review the 'for' loop statement, learn how to use variables to count information within a loop, understand the increment operator 'i++' and decrement operator 'i--'.
6.Learn how to use 'for' loops for multiple inputs and their applications.
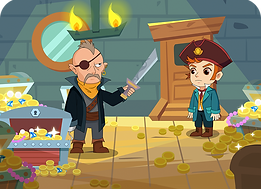.png)
Unit 02
7 Lessons
118 Challenges
1.Review 'if' statements and 'for' loops, learn the method of using 'for' statements and 'if' statements in parallel in the program.
2.Learn how to nest 'if' statements in 'for' loops, and nest 'for' statements in 'if' statements.
3.Learn how to use variables to count information in nested 'for' and 'if' statements.
4.Learn the usage of 'break' and 'continue' in loops.
5.Learn arrays, and methods for inputting and assigning values to arrays.
6.Learn how to use variables to count array information.
.png)
Unit 03
7 Lessons
100 Challenges
1.Understand decimals, learn the 'double' variable type, and acquire the ability to convert 'double' to 'int' and vice versa in programs.
2.Explore the variation of variables in loops, and further study the usage of accumulation.
3.Learn methods to iterate through arrays and perform operations on each element.
4.Study 'if...else if...else' statements, and learn to evaluate multiple conditions.
5.Review 'for' loops and 'if...else' statements, and understand the difference between parallel 'if' statements and 'if...else if' statements.
6.Learn nested 'if' statements, and acquire the skill to use nested 'if' statements to solve complex logical problems.
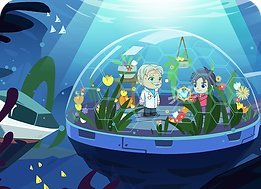.png)
Unit 04
7 Lessons
111 Challenges
1.Introduction to logical operators, using "and" and "or" to simplify complex logic into simple conditional statements.
2.Understanding characters, learning and using char type variables, understanding ASCII codes and the correspondence between characters, and learning to convert between uppercase and lowercase letters.
3.Understanding strings, learning and using string type, understanding the usage of '.length()', and learning methods to iterate through strings.
4.Learning 'while' loops and their applications, solving problems like digit sum calculation.
5.Introducing bool type and its relationship with logical operators, learning to use bool to determine if n conditions are true.
6.Understanding the difference between global variables and local variables, and learning the meaning and usage scenarios of arrays with meaningful contents.
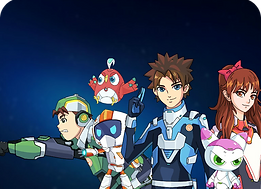.png)
Unit 05
7 Lessons
99 Challenges
1.Understand the meaning of nested 'for' loops, use nested 'for' loops to solve simple practical problems.
2.Introduce common problems that require two layers of nested 'for' loops to solve, learn to repeatedly find the minimum or maximum value within a range.
3.Recognize and learn to analyze the structure of complex programs, use output debugging to solve bugs in programs.
4.Dive deeper into nested 'for' loops, learn to use the outer loop variable to control the number of iterations of the inner loop.
5.Understand functions, learn and use void functions that do not return value.
6.Introduce function return values and their applications, learn to reduce repetitive code segments using functions.
7.Learn the increment and decrement operators.
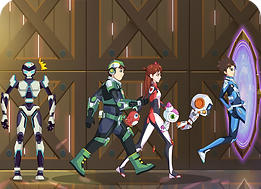.png)
Unit 06
7 Lessons
94 Challenges
1.Learn to use 'continue' and 'break' to control the execution order of loops in nested loop programs.
2.Delve into functions and nested loops, optimize the inner loop using functions.
3.Study the principles of sorting algorithms, and learn to write simple sorting algorithm programs.
4.Learn bubble sort and counting sort, and compare the differences between various sorting algorithms.
5.Understand the concepts of function libraries and namespaces, master common functions in C++.
6.Understand the definition, input, output, and traversal of two-dimensional arrays, and use two-dimensional arrays to solve practical problems.
7.Learn and use the basic mathematical functions 'abs()', 'ceil()', 'floor()', 'round()', and become familiar with their functions.
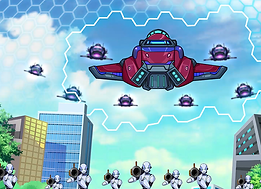.png)
Unit 07
7 Lessons
76 Challenges
1.Understand time complexity, learn about coefficients, exponents, calculation methods, common code scenarios, and time limits.
2.Understand prefix sum arrays, master the method to quickly calculate the sum of intervals using prefix sum arrays.
3.Understand binary search, grasp its implementation, and learn to use binary search to solve practical problems.
4.Review the principles of binary search.
5.Understand the concept and execution process of recursion, and be able to use recursive thinking to solve practical problems.
6.Learn the concept of memoization search, and be able to write recursive functions using this concept.
.png)
Unit 08
7 Lessons
70 Challenges
1.Review the principles of binary search algorithm and complete advanced practice exercises.
2.Learn recursive algorithms.
3.Master solutions for problems like derangement problem and balls into boxes problem.
4.Further study of binary search algorithm, understand its application in real number ranges, and grasp the differences between binary search in real numbers and integers.
5.Understand concepts such as Sieve of Eratosthenes, prime factorization, and factorial decomposition.
6.Review Sieve of Eratosthenes, continue advanced practice exercises focusing on binary search algorithm.
7.Review and reinforce binary search algorithm and iterative algorithms, learn the applications of 'lower_bound' and 'upper_bound' functions.